1.0 Python – Chapter 1 – print and input
There are currently 4 functions we should all be familiar with:
- input
- int
- str
We should also be familiar with the concept of a variable.
print is used to display output on the screen. For example:
>>>print("Hello, world!") Hello, world!
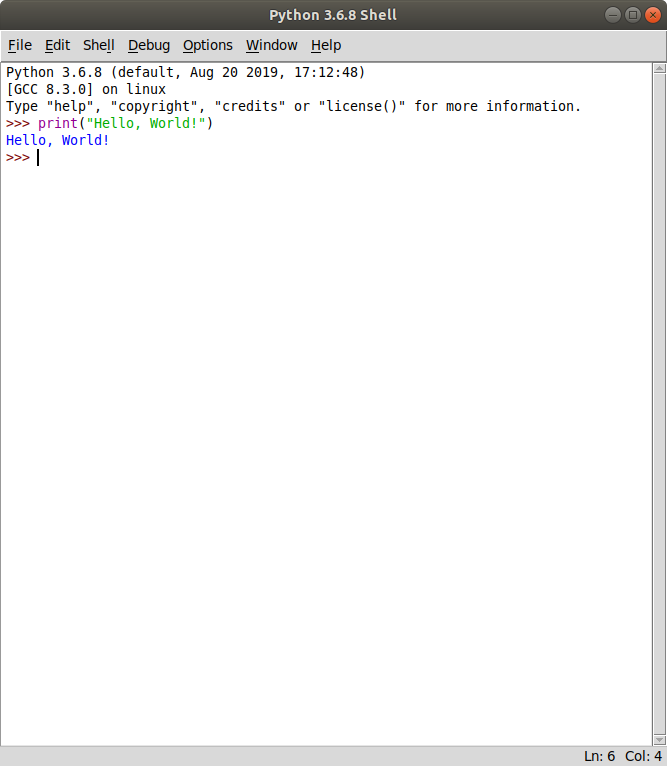
input
input is used to get information from a user. For example:
MyVar=input("Enter some information: ")
In the input example above, MyVar would hold the string representation of what the user typed in.
After learning the basic functions for input and output we learned to write a simple program that uses both of these functions:
YourName=input("What is your name? ")
print("Hello "+YourName)
The two lines of code above when executed will ask the user for their name and then display “Hello + The Users Name”. ie:
What is your name? Mr. Campbell
Hello Mr. Campbell
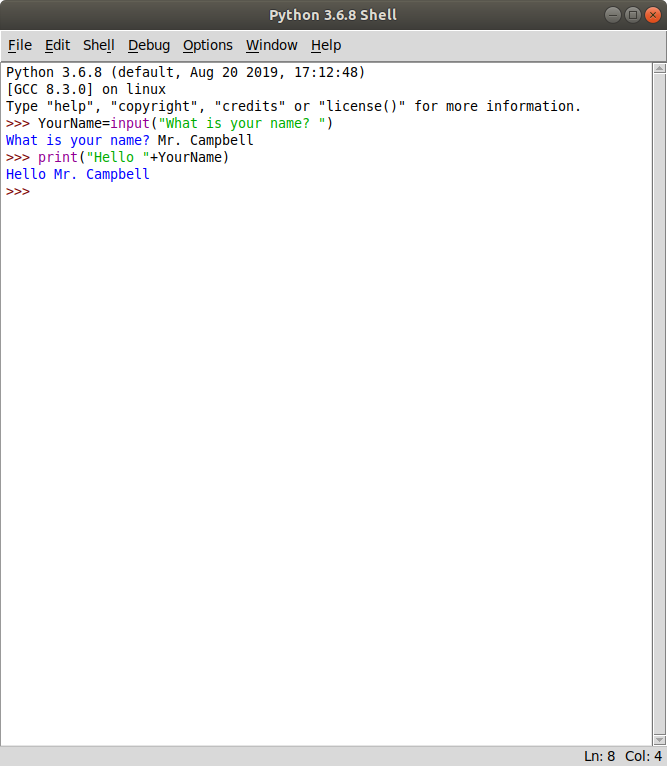
In the second line of code (“Hello ” + YourName) is the argument we pass to the print function. The print function sees this as one string. The + sign joins the two strings together. This is called concatenation.