Controlling a Motor
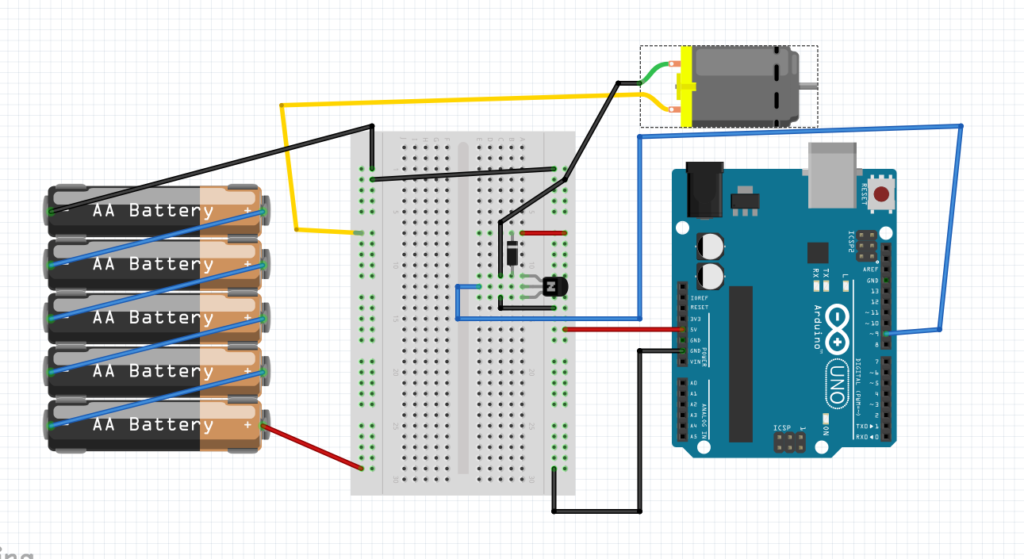
Pictured above is a diagram for connecting a motor to an Arduino. The Motor in our example is connected to digital pin 9. The following code will cause the motor to speed up and slow down.
/*Motor Speed Demonstration
* This program demonstrates adjusting the speed of a
* Motor connected to digital pin 9
*/
/*Pin Motor is connected to*/
int Motor1 = 9;
// the setup routine runs once when you press reset:
void setup() {
// declare pin 9 to be an output:
pinMode(Motor1, OUTPUT);
}
/*SetMotorSpeed is a function that uses the
*analog write function to set the speed of the
*Motor. It takes two arguments, the pin#
*and the speed (0 - 255)
*/
void SetMotorSpeed(int Motor,int Speed){
analogWrite(Motor,Speed);
}
// the loop routine runs over and over again forever:
void loop() {
//This first for loop incrementally increases
//The Speed
for(int Speed1=50;Speed1<256;Speed1++){
SetMotorSpeed(Motor1, Speed1);
delay(50);
}
//this for loop decreases the speed
for(int Speed2=255;Speed2>50;Speed2--){
SetMotorSpeed(Motor1, Speed2);
delay(50);
}
}