1.2 Python – Chapter 1 – str()
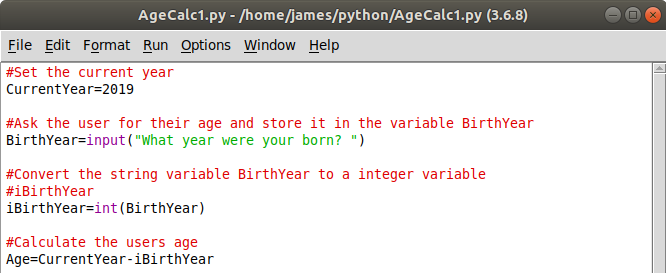
In our last example, pictured above, we calculated the users age. We asked for the year they were born using the input() function, converted the value the user entered from a string to an integer using the int() function, and then calculated the users age by subtracting the users birth year from the current year.
Remember: BirthYear, which we get from the input() function, is a string value. input() returns a string. iBirthYear is an integer. we used the int() function to convert the string BirthYear to the integer iBirthYear.
str()
Now it is time to let the user know the result of our calculation. We cannot concatenate an integer to a string. We will use the str() function to convert the calculated age to a string to use for our output.
#Set the current year
CurrentYear=2019
#Ask the user for their age and store it in the variable BirthYear
BirthYear=input("What year were your born? ")
#Convert the string variable BirthYear to a integer variable
#iBirthYear
iBirthYear=int(BirthYear)
#Calculate the users age
Age=CurrentYear-iBirthYear
#Convert the age to a string
sAge=str(Age)
#Concatenate the text of our output and the users age
YourAge="Based on our calculations, you are " + sAge +" years old!"
#Display our result to the user
print(YourAge)
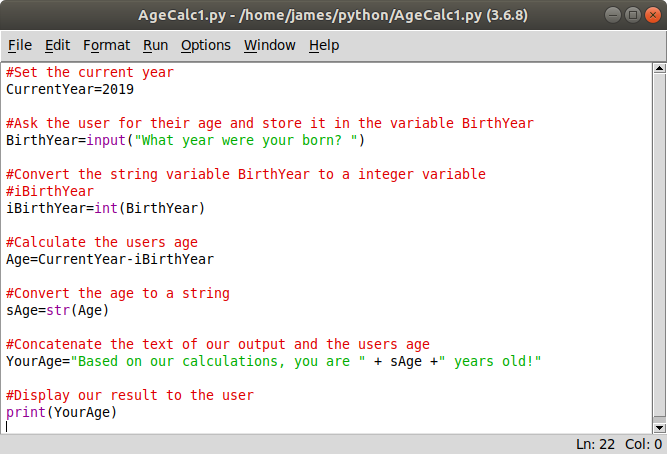
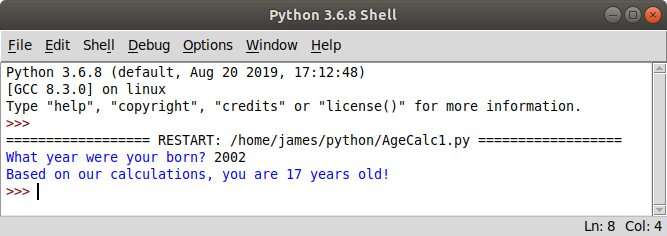