1.1 Python – Chapter 1 – int()
When working in Python we store data in variables. Variables are containers where data can be stored in memory. We set and retrieve the information stored in a variable by using the variables name. To set information in a variable we use the assignment operator =
x=8
In the code above the variable name is x and we assigned it a value of 8.
MySchool="Kellenberg Memorial High School"
MySchool is another variable name and we have assigned it the value “Kellenberg Memorial High School”
Python does not require us to tell it what type of data is being stored in the variable. In our examples above x is an integer type and MySchool would be a string type.
We Know the x is an integer value because 8 is a number that is not wrapped in single or double-quotes. MySchool is a string because it is wrapped in double-quotes. If we attempted to assign a string to a variable without quotes we would get an error.
int()
The input function returns a string:
YourName=input("What is your name? ")
In the example above the variable, YourName is a string value that is returned from the input function.
If we wanted to figure out a persons age based on the year they were born we would have to convert the return value from the input function to an integer. To convert a string to an integer we use the int() function.
The int() function takes a string as an argument and returns an integer. In the example that follows we ask the user for what year they were born in and calculate their age.
#Set the current year
CurrentYear=2019
#Ask the user for their age and store it in the variable BirthYear
BirthYear=input("What year were your born? ")
#Convert the string variable BirthYear to a integer variable
#iBirthYear
iBirthYear=int(BirthYear)
#Calculate the users age
Age=CurrentYear-iBirthYear
Now that we have the users age as an integer value and want to display it. We will use the print() function, but we have to concatenate the user’s age with a string message, such as “Your age is: “
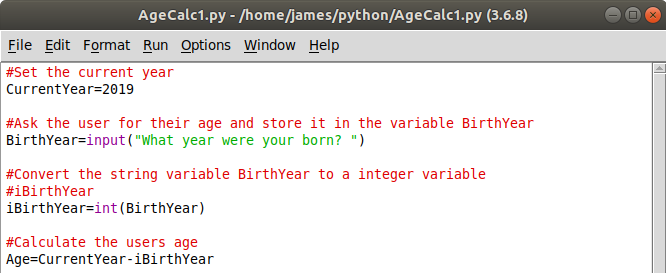
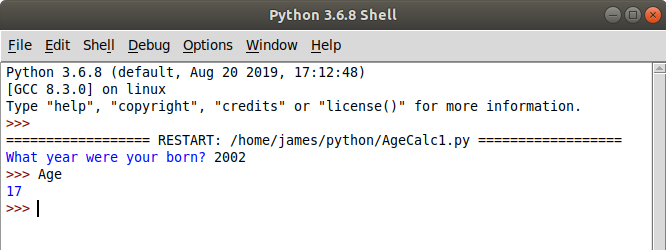